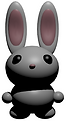
Learn Computer Science
Developing a more interesting World
"Computer science is no more about computers than astronomy is about telescopes."
Edsger Dijkstra
Free beginner - intermediate level computer science tutorials
Conditionals
Tutoring > Java Coding > Conditionals
There are several conditional statements we can use to direct the flow of control in our code. For example, we may want to do something only if something else is true, or if a certain number is greater than a certain value. We will go through each conditional with examples.
The If Statement
If statements execute whatever is in the curly braces {} if and only if whatever is in the parentheses () equates to true. The general syntax for an if statement is as follows:
if (what we want to test) {
// do something here if what we want to test is true.
}
So an example could be:
if (applesCount < 10) {
System.out.println ("There are less than 10 apples");
}
This will print "There are less than 10 apples" if and only if the value of the variable applesCount is less than 10.
We can give an alternative outcome by using the else keyword. If the conditional statement equates to false, the second message is printed:
if (applesCount < 10) {
System.out.println ("There are less than 10 apples");
} else {
System.out.println ("There are 10 or more apples");
}
If, and else if
We can also use else if to test for a second condition and perform the appropriate action if it equates to true. Remember that the code will exit the loop if the first condition is true so wil not reach the else if.
if (applesCount < 10) {
System.out.println ("There are less than 10 apples");
} else if (applesCount > 10) {
System.out.println ("There are more than 10 apples");
} else {
System.out.println ("There are 10 apples");
}
The while loop and do-while
The while loop continues executing whatever is inside the curly braces {} as long as the condition in the parentheses remains true.
while (this is true) {
// do this
}
If the condition is not true when the while loop is reached, it will just be ignored and execution will continue on down the code after the loop.
while (appleCount < 10) {
appleCount++;
}
This will continue to add one apple at a time until appleCount reaches 10. Note that ++ means "increment by one". Similarly -- means "decrement by one".
Do while is the same but will always execute the loop at least once, regardless of whether the conditional is true or not. The condition is evaluated after the statement has executed once:
do {
statement(s)
} while (expression);
The for loop
A for loop (or for each loop) will evaluate something and do something for every true condition. The syntax is as follows:
for ( initialisation ; termination ; increment ) {
statement(s)
}
-
The initialisation is a variable you create (conventionally an integer called "i"). This initialises the loop.
-
When the termination expression evaluates to false, the loop terminates.
-
The increment is executed at the end of each iteration of the loop. This can either be ++ or --.
For example:
for ( int i = 0 ; i < = 100 ; i++ ) {
System.out.println( "Count is:" + i );
}
This will iterate through 101 times (including the 0th time) and add one to i each time until i equals 100. Each time it iterates through the loop it will print out the message "Count is:" followed by the current value of i. So the output we get will be something like this:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
... and so on until ...
Count is 100
The switch statement
The switch statement can have multiple different outcomes depending on which case is true. Here is the syntax:
switch (expr) { case c1: statements // do these if expr == c1
break;
case c2: statements // do these if expr == c2
break;
case c2:
case c3:
case c4: // Cases can simply fall through.
statements // do these if expr == any of c's
break;
. . .
default: statements // do these if expr != any above
}
Note that we must write break in order to exit the statement. The body of a switch statement id called the switch block. In here we can write any number of different cases which, if true, execute the corresponding code. If the cases all fall through, the default section is executed.
Here is an example:
int day = 3; // We initialise an int variable to 3 here as an example.
String output; // This will be the output.
switch (day) {
case 1: output = "The day is Monday";
break;
case 2: output = "The day is Tuesday";
break;
case 3: output = "The day is Wednesday";
break;
case 4: output = "The day is Thursday";
break;
case 5: output = "The day is Friday";
break;
case 6: output = "The day is Saturday";
break;
case 7: output = "The day is Sunday";
break;
default: output = "Invalid day number!"
}
The output here will be "The day is Wednesday" as case 3 evaluates to true.