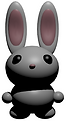
Learn Computer Science
Developing a more interesting World
"Computer science is no more about computers than astronomy is about telescopes."
Edsger Dijkstra
Free beginner - intermediate level computer science tutorials
Variables and Types
Tutoring > Java Coding > Variables and Types
A variable is like a container that holds a type of data. Java is a strongly typed language, which means every variable has a data type associated with it. When we create a variable we allocate memory depending on which type we create.
There are 8 primitive types in Java, they are as follows:
For numbers:
-
int - this is for integral numbers, which are whole numbers. It allocates 32 bits of memory for a positive or negative whole number.
-
long - same as an int, but allocates 64 bits of memory.
-
short - same as an int, but allocates 16 bits of memory.
-
byte -same as an int, but allocates 8 bits of memory.
-
float - this is for floating point numbers (numbers with a decimal place). It allocates 32 bits of memory.
-
double - this is the same as float but allocates double the amount of memory (64 bit).
For characters/other:
-
boolean - this can either be true or false. Use it for simple flags that track true/false conditions.
-
char - holds a single 16 bit unicode character. We write a char using single quotes like this: 'a'
There are other types of variable but we will not look at these here.
Another common type is String. String is actually an object and it allows a string of characters to be stored. Basically everything between the two double quotes is the string. For example, we could initialise a String variable like this:
private String name = "Ted";
Member and local variables, and parameters
A global variable is declared in a class outside any method, these are members of their class. They are also called field variables. They are accessible to the whole class.
Local variables are declared within the curly brackets {} of methods and are only accessible to their respective method.
A parameter is a variable holding a value we want to pass into a method. More on this in Methods.
Access modifiers and declaring variables
In order to use variables we need to first declare them. We do this by first writing an access modifier (public, protected or private). Simply, public means that the variable can be accessed from anywhere, private means it can be accessed from only within the current class. Protected means package access, anything in the same package
Next we write the type of the variable we want, followed by a name we choose for it (the name is also referred to as the identifier). We can choose any name we like as long as it isn't the same as any Java reserved keywords. Also, it cannot start with a digit, but can contain digits after the first letter. You also cannot use spaces or other symbols.
Conventionally, we should also use a lowercase letter to begin the name and a capital letter for any further words in the name. This is known as CamelCase. For example: carColour, or accountBalance.
The name of variables should describe what they represent, it's better to have a longer name if it is less ambiguous than a shorter one.
Initialising variables
In order to use variables we initialise them by giving them a value. We use the assignment operator (the equals sign: = ) along with a value of the same type:
private int myInt = 15;
We can initialise a variable when we declare it or later on, but we must initialise it before we can use it.
Static and final variables
We can declare a variable to be static or final.
A static variable does not need an instance of a class (an object) in order to be used.
When a variable is declared final, it is a constant and cannot be changed from it's initial value. Final can also be used for classes or methods.