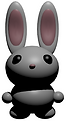
Learn Computer Science
Developing a more interesting World
"Computer science is no more about computers than astronomy is about telescopes."
Edsger Dijkstra
Free beginner - intermediate level computer science tutorials
Methods
Tutoring > Java Coding > Methods
We can perform operations on variables and objects by using methods in Java. A method is simply a portion of code which performs some action and returns a value to it's caller. The caller of the method is the piece of code that calls or "invokes" the method, basically saying "execute the code inside this method and then return to the same place in the code from where we called it".
The parts of a method are:
-
An access modifier - public, protected or private. More on access modifiers in Variables and Types.
-
The return type - we specify what type of value is returned to the caller when the method has finished executing. If we don't actually want to return a value, we can just write void, this means literally nothing, not zero, nothing.
-
The method name - can be any legal identifier, however convention dictates we should start with a lower case letter and is usually a verb describing what the method does, such as calculateAnswer or addNumbers.
-
Parentheses () - these can be empty, or otherwise they hold what we call parameters. These are arguments that we "pass into" the method in order to perform operations.
-
The method body - enclosed between curly braces {}.
An example method is shown here:
public int addFruits(int appleCount, int orangeCount){
int sum = appleCount + orangeCount;
return sum;
}
Here the method name is addFruit, the method is public and we are returning an integer. The method takes 2 parameters, appleCount and orangeCount and in the method body (between the {}) we declare an int variable called "sum" and assign the sum of the two parameters to it. We then return the integer "sum".
So what this method does is it takes two numbers (the appleCount and orangeCount) and adds them together, and returns the result.
What we would do is define this method somewhere in one of our classes and when we want to use it, we call it or invoke it by just typing it's name, followed by the required values in the parentheses like this:
addFruits(4, 7);
So this gives the appleCount variable the value 4, and the orangeCount variable 7, and passes them into the method. The method adds them together and returns the integer value 11.
This is just a simple example, methods become really useful when we want to perform complex operations on data and are great for doing something repeatedly as once you have defined a function, you just have to type the name as above. Note that the number of parameters a method requires is the number you have go provide (even zero) and they must be of the correct type, you can't pass a double when an int is required).
Constructors and the main method
The main method is the entry point for a program, this is what the compiler looks for when it starts executing a program. The parameters or "arguments" supplied to it is an array of Strings called args, These are any Strings written in the command line when you run the program, you don't have to write any but the option is there. This is what the main method looks like:
public static void main(String [] args){
//write what you want to happen in the method body
}
We generally want to write most of our code outside of the main method and just make calls to classes and methods containing operations we need.
A constructor of a class actually creates an object instance of that class. It is like a method but has the same name as the class it is to construct and has no return type. For example, if you has a class called "Product" a possible constructor could be this:
public Product(){
}
This would create an instance of the Product class. we do this by using the new operator ilke this:
Product myProduct = new Product();
More on object creation in Objects.